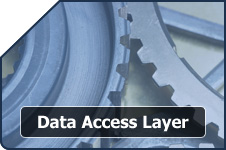 | Home > Application Framework > Data Access Layer
The Data Access Layer in the StrataFrame Application Framework is stateless, highly
cohesive, and loosely coupled. Unlike other application frameworks, or data access
layers generated by O/R Mappers, a single class is used for all data access within a
StrataFrame application. The developer does not interface directly with the
DataAccessLayer class, but rather indirectly through the BusinessLayer.
| 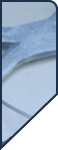 |
Overview
A data access layer should be fast, efficient, and transparent to the application developer;
StrataFrame’s DataAccessLayer is engineered to meet each of these
criteria. This single class houses the data access
logic for the entire framework. Since it remains stateless and loosely coupled, it is used by all
business objects as a bridge to access the data store. The data access layer is provider
independent (SQL Server, Oracle, or OLEDB), and it leverages many optimization features from the
different providers that ensure delivery of excellent performance.
- Supported Databases
- SQL Server 2000
- SQL Server 2005
- SQL Server 2008
- Oracle
- OLEDB
| - Data Access Layer Anatomy
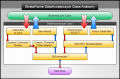
|
StrataFrame natively supports SQL Server, Oracle, Visual FoxPro, and
Microsoft Access.
Additionally, any OLE DB provider can be used
to connect to the data through the data access layer.
Stateless, Highly Cohesive, and Loosely Coupled
StrataFrame’s data access layer has been designed to be stateless, highly cohesive, and loosely
coupled. Its statelessness allows all business objects to utilize an instance of the same
DataAccessLayer class rather than requiring a specific implementation of a data access layer
class for each business object. The data access layer is completely separated from the business
layer and performs only the functions specifically required by a data access layer.
Transparent to the Application Developer
By housing an instance of the DataAccessLayer class within each business object instance, the
data access layer becomes completely transparent to the application developer. The developer never
calls upon it directly but rather indirectly through factory and data retrieval
methods within a business object.
Streamlined Data Access
Improvements achieved in ADO.NET 2.0 have allowed the data access layer to become completely
streamlined, efficient, and optimized. Additionally, the data access layer takes advantage
of asynchronous processing, enabling it to commit records to the data store across multiple
threads. By threading the data access, application latency is reduced, particularly across
a VPN or remote connection.
Full Concurrency Support
The DataAccessLayer class contains core level support for concurrency and offers the
developer three choices:
None |
This setting will bypass all concurrency record handling.
| Optimistic |
This technique directly follows Microsoft’s definition of optimistic
concurrency. (See MSDN
for additional information)
| Row Version |
This mode allows a specific field to be specified for row versioning purposes. This
is the most optimized method of concurrency support since it requires the fewest
number of tests on the server to determine if a soft collision potentially exists.
When a row is saved, the DataAccessLayer automatically updates the row version. | Time Stamp |
Using a time stamp field is similar to using the Row Version method. When this option
is used, a TIMESTAMP column is specified as the row versioning key instead of an
integer-based column. | Collision Notification Options
When a concurrency exception is detected by the framework, the DataAccessLayer retrieves the conflicting
record from the database and compares the local version of the record to the server of the record. If
no mismatched data is found, the record is forced to save, and the end-user is never notified of the
data collision. If mismatches are found, then the end-user is notified that someone else modified the record
first and the end-user is presented with a form that allows them to correct the concurrency issues before
saving the record again.
This auto-handling of data collisions can be disabled, and there are properties that determine how
the developer will be notified if a concurrency exception occurs. By default, an event is raised
that is automatically handled by the business object's parent form (to present the end-user with the
list of mismatched values), but a business object can be configured
to throw an exception when a collision occurs.
Synchronized and Thread-Safe
The DataAccessLayer class has been synchronized to prevent unwanted repercussions stemming
from simultaneous access by multiple threads. Requests for data can be made by the business
layer on different threads without requiring the developer to explicitly synchronize those
requests. Furthermore, events raised by the data access layer are explicitly raised on the
main application thread to prevent cross-thread exceptions as the events are bubbled
to the user interface.
Advanced CRUD (Create, Update, and Delete) SupportStored Procedures
CRUD settings are critical when attempting to achieve the most optimized and streamlined
insert, update, and delete performance between the DataAccessLayer and the database.
In some cases, company policy forces a developer
to use stored procedures on all CRUD interaction. In these situations the CRUD options are not
only helpful; they are vital. A stored procedure can be specified for each CRUD operation (INSERT, UPDATE, and DELETE) through
properties on the business object, which uses those settings in turn when interacting with the DataAccessLayer.
Primary Key Value Auto Retrieval
When a new record is created, the primary key value(s) are automatically retrieved and updated within the
internal data source of the business object. This feature can be adjusted through the CRUD settings as well.
Unlimited Server Connections and Database TypesUnlimited Server Connections
Oftentimes a developer may have more than one data source that
will be viewed, updated, and deleted. This is especially common when interacting with disparate or legacy data.
Therefore, a StrataFrame application allows an unlimited number of connections that can be accessed within
the application simultaneously.
The separate connection strings needed for each connection are
managed by the Connection String Manager provided by StrataFrame.
Multiple Database Type Support
Expanding upon the unlimited server connections is the capability to connect to an unlimited number of databases
at the same time. This includes different database types such as SQL Server and FoxPro.
This functionality is significant because it allows business objects to communicate to SQL Server and FoxPro databases,
for example, on the same form, and the developer does not have to manage these connections. This
is an advantageous means of migrating existing legacy applications forward incrementally, or of creating
streamlined data conversions.
Extensive Data Retrieval MethodsCustom SELECT Statements
Unlike many other frameworks and O/R Mappers, a custom SELECT statement or stored procedure can be easily executed for data retrieval. The developer is not limited to the stored procedures and pre-generated SELECT statements used by the data access layer.
Parameterized Commands and Stored Procedure Queries
Another useful data retrieval option is a DbCommand. This is the best data retrieval option when creating
dynamic queries or when a WHERE clause will be stated, since the DbCommands have parameter support. A parameterized
DbCommand can also call a stored procedure.
Non-Database Specific Queries
There are circumstances where the backend database may change from one type to another depending
upon the deployment environment. The StrataFrame QueryInformation allows developers to create provider-independent queries
that are converted to the appropriate provider-specific SQL syntax by the database's specific DbDataSourceItem
implementation. This
powerful feature allows a single query to be written and re-used to query SQL Server, FoxPro, Oracle, or any
database type being used by the application, as long as there is consistency between the data
structures.
Scalar Support
StrataFrame has full scalar support, which allows a developer to quickly retrieve a single piece of data or
to execute a query that performs a simple calculation and returns the calculated value.
Transaction ProcessingUnlimited Simultaneous Transactions
StrataFrame takes complete transaction support to a new level by allowing an unlimited
number of transactions to be processed at the same time. Transaction objects are managed by the framework
allowing a "hands-free" approach to transaction processing.
Each transaction can be individually controlled, and all business objects have the
ability to be included or excluded from the transaction. This can be very beneficial when a large transaction
is being processed and the developer wants to save data
from another business object on a separate transaction or off a transaction entirely.
Simple Programmatic Access
The transaction processing can be control through very simple and accessible methods and is as simple as
calling TransactionBegin() along with TransactionEnd() or TransactionRollBack(). Any errors or notifications that need to be managed
will be automatically presented to the developer, who then must simply decide which action to take.
Data Debug Mode
The debug mode provides a complete view of each SQL statement executed on a data source, including
SELECT, INSERT, UPDATE, and DELETE statements. Each statement is written to a log file in
HTML format showing the SQL syntax, parameters, transaction context, connection,
and many other pieces of information.
This feature is invaluable when trying to track down
any type of error stemming from data processing that cannot be easily debugged through Visual Studio.
Technical Specifications & Featuresexpand all | collapse all | |
 | Dynamic Command Builder | | | |
Commands used by the DataAccessLayer class to persist data to the data store
are built dynamically. This allows the data access layer to remain stateless
and improves performance by customizing the command to the record/table being
persisted.
| | |
|  | Insert/Update Stored Procedures Availability | | | |
The data access layer supports the use of stored procedures for INSERTs and
UPDATEs. When the Application Framework is used in conjunction with the
Database Deployment Toolkit, these stored procedures are automatically
generated and registered.
| | |
|  | Custom SELECTs & Stored Procedures for Retrieval | | | |
Unlike many other frameworks and O/R Mappers, a custom SELECT statement or
stored procedure can be easily executed for data retrieval. The developer
is not limited to the stored procedures and pre-generated SELECT statements
used by the data access layer.
| | |
|  | Parameterized Commands | | | |
All dynamically built commands are parameterized. Parameterization improves
the execution performance of commands on the data store and alleviates type
mismatches and problems with delimiters, such as quotes within VARCHAR values.
| | |
|  | Transparent to the Developer | | | |
The data access layer within StrataFrame is transparent to the application
developer. A developer is never required to directly interact with a DataAccessLayer
object.
| | |
|
| expand all | collapse all | |
 | Transaction Processing | | | |
StrataFrame gives the developer full control over non-distributed (non COM+)
transaction processing. A single transaction can span multiple databases and
any business object can be saved on the transaction. If the transaction is
rolled back for any reason, the business objects that participated in the
transaction will be rolled back as well.
| | |
|  | Connection String Management | | | |
The StrataFrame Application Framework provides a complete interface for the
management of connection strings. While most application frameworks require
that the connection string be embedded within the application or stored in a
clear-text format on the client machine, StrataFrame allows the developer to
store the connection string in an encrypted file on the server. Through the
provided user interface, an end-user can quickly set up or change his or her
connection string. The connection string management also supports real-time
connection string changes, a network shared settings file, and multiple
connection strings per application.
| | |
|  | SQL Server 2005 Query Notifications Support | | | |
Building upon new functionality provided by SQL Server 2005, the data access
layer supports Query Notification Services. When configured (by default), the
data access layer will automatically register a query notification with each
SELECT statement executed for data retrieval. Upon receiving a notification
from the server, the DataChanged event is bubbled up to the business layer for
processing.
| | |
|  | Multi-Threaded Updates | | | |
StrataFrame’s data access layer takes complete advantage of asynchronous
processing provided by many data stores (e.g., SQL Server 2005, Oracle 10g). This
asynchronous processing allows the DataAccessLayer class to update records
from a business object across multiple threads thereby increasing speed
dramatically.
| | |
|
|
|